Multidimensional Array
A multidimensional array is an array with many dimensions. This is a multi-level array; a multilevel array. The 2D array or 2D array is the simplest multi-dimensional array. As you will see in this code, this is technically an array of arrays. A 2D array or table of rows and columns has sometimes called a matrix.
The multi-dimensional array declaration is identical to a one-dimensional array. We have to say that we have 2 dimensions for a 2D array.
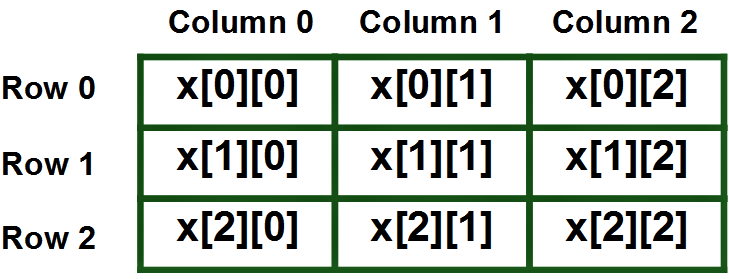
A twin-dimensional array or table can be stored and retrieved with double indexation like a one-dimensional array (column rows) (array[row][column] in typical notation). The number of indicators required to specify an element is termed the array dimension or dimension. int two_d[3][3];
C++ Multidimensional Arrays
The multi-dimensional array has sometimes referred to as a C++ rectangular array. Two- or three-dimensional may be available. The data has kept in a tabular form (row/column), often referred to as a matrix.
C++ Multidimensional Array Example
Let’s examine a basic C++ multi-dimensional pad, where two-dimensional pads are declared, initialized, and crossed.
- #include <iostream>
- using namespace std;
- int main()
- {
- int test[3][3]; //declaration of 2D array
- test[0][0]=5; //initialization
- test[0][1]=10;
- test[1][1]=15;
- test[1][2]=20;
- test[2][0]=30;
- test[2][2]=10;
- //traversal
- for(int i = 0; i < 3; ++i)
- {
- for(int j = 0; j < 3; ++j)
- {
- cout<< test[i][j]<<” “;
- }
- cout<<“\n”; //new line at each row
- }
- return 0;
- }
Multidimensional Array Initialization
Like a regular array, a multidimensional array can be initialized in more than one method. Initialization of two-dimensional array:
int test[2][3] = {2, 4, 5, 9, 0, 19};
A multidimensional array can be initialized in more than one method.
int test[2][3] = { {2, 4, 5}, {9, 0, 19}};
This array is divided into two rows and three columns, and we have two rows of elements with every of three elements.
Initialization of three-dimensional array
int test[2][3][4] = {3, 4, 2, 3, 0, -3, 9, 11, 23, 12, 23,
2, 13, 4, 56, 3, 5, 9, 3, 5, 5, 1, 4, 9};
The value of the first size is 2. Thus, the first dimension comprises two elements:
Element 1 = { {3, 4, 2, 3}, {0, -3, 9, 11}, {23, 12, 23, 2} } Element 2 = { {13, 4, 56, 3}, {5, 9, 3, 5}, {5, 1, 4, 9} }
In each of the components of the second dimension, there are four int numbers:
{3, 4, 2, 3} {0, -3, 9, 11} ... .. ... ... .. ...
Example 1: Two Dimensional Array
// C++ Program to display all elements
// of an initialised two dimensional array
#include <iostream>
using namespace std;
int main() {
int test[3][2] = {{2, -5},
{4, 0},
{9, 1}};
// use of nested for loop
// access rows of the array
for (int i = 0; i < 3; ++i) {
// access columns of the array
for (int j = 0; j < 2; ++j) {
cout << "test[" << i << "][" << j << "] = " << test[i][j] << endl;
}
}
return 0;
}